In this tutorial how to insert data in a MySQL table using PHP.
You can do this with the INSERT INTO statement. There are three ways we can write this.
- Procedural Method
- Object Oriented Method
- PDO Method
We will discuss all the methods here. You can use any one.
PHP MySQL INSERT Query
We will discuss Procedural Method. Example is given below.
$con = mysqli_connect("localhost", "database user", "database Password", "database name"); ?>;
First, we will create a database connection. $con is a variables. mysqli_connect() function is establish database connection. It is always connected localhost. This function requirement database user, Password and database name.
if($con === false){ die("ERROR: Could not connect. " . mysqli_connect_error()); } ?>;
This upper PHP code is checking. Database connection has been made. If not, then it will give the error. mysqli_connect_error() is function give the error.
$sql = "INSERT INTO member (name,addr,email) VALUES ('saurav', 'kolkata', '[email protected]')"; ?>;
INSERT INTO is a PHP query. This PHP query insert value database table. MEMBER is a table name and name,add,email is a table Field name. $sql is a PHP variables. Database structure example is given below.
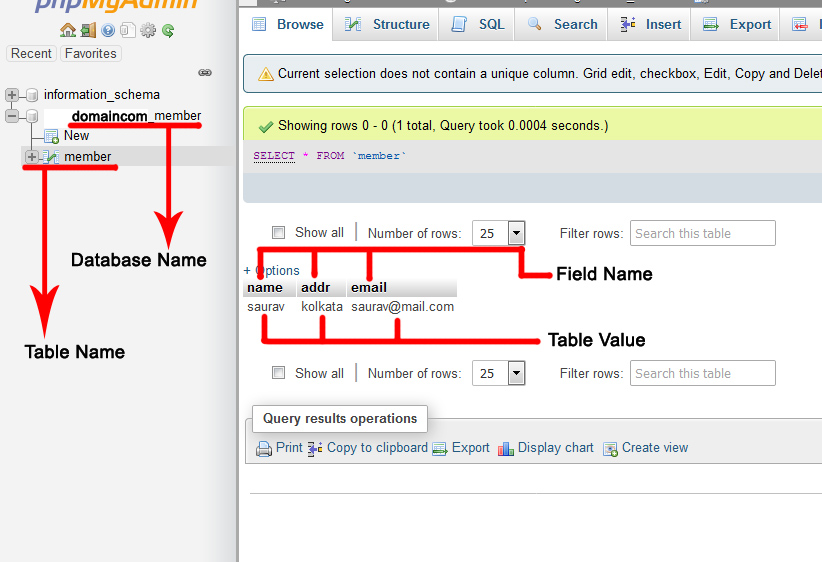
if(mysqli_query($con, $sql)){ echo "Records inserted successfully."; } else{ echo "ERROR: Could not able to execute $sql. " . mysqli_error($con); } // Close connection mysqli_close($con); ?>;
This mysqli_query() execute INSERT INTO PHP query. This mysqli_query() can’t execute INSERT INTO PHP query. Show error “Could not able to execute”. It is if and else condition. Ending Close this connection. Full code given bellow.
//Database connection $con = mysqli_connect("localhost", "database user", "database Password", "database name"); // Check connection if($con === false){ die("ERROR: Could not connect. " . mysqli_connect_error()); } // Attempt insert query execution $sql = "INSERT INTO member (name,addr,email) VALUES ('saurav', 'kolkata', '[email protected]')"; if(mysqli_query($con, $sql)){ echo "Records inserted successfully."; } else{ echo "ERROR: Could not able to execute $sql. " . mysqli_error($con); } // Close connection mysqli_close($con); ?>;
How to use this PHP code in HTML
Creating the HTML Form
This is a simple HTML form. This form gives you three input.
Untitled Document
Creating insert.php Page
<?php $name=$_REQUEST["name"]; $addr=$_REQUEST["addr"]; $details=$_REQUEST["details"]; $email=$_REQUEST["email"]; $con = mysqli_connect("localhost", "database user", "database Password", "database name"); // Check connection if($con === false){ die("ERROR: Could not connect. " . mysqli_connect_error()); } // Attempt insert query execution $sql = "INSERT INTO member (name,addr,email) VALUES ('$name', '$addr', '$email')"; if(mysqli_query($con, $sql)){ echo "Records inserted successfully."; } else{ echo "ERROR: Could not able to execute $sql. " . mysqli_error($con); } // Close connection mysqli_close($con); ?>
When user clicks the submit button in the HTML form. The form data is sent to insert.php file. The insert.php file connect to the MySQL database server and insert value database member table.
The next tutorial insert data Object Oriented Method and PDO Method